
Sunday, December 21, 2008
Wednesday, November 12, 2008
Thursday, October 2, 2008
Rails Hello World!
App\Controllers\say_controller.rb
class SayController < ApplicationController
def hello
@time = Time.now
end
def goodbye
end
def files
@files = Dir.glob('*')
end
end
app\views\say\hello.html.erb
<html>
<head>
<title>Hello, Rails!</title>
</head>
<body>
<h1>Hello from Rails!</h1>
<ul>
<li>Addition: <%= 1+2 %> </li>
<li>Concatenation: <%= "cow" + "boy" %> </li>
<li>Time in one hour: <%= 1.hour.from_now %> </li>
</ul>
<% 3.times do %>
Ho!<br />
<% end %>
Merry Christmas!
<br /><br />
<% 3.downto(1) do |count| -%>
<%= count %>...<br />
<% end -%>
Lift off!
<br /><br />
Email: <%= h("The <thekhuc@gmail.com>") %>
<p>
It is now <%= Time.now %>
</p>
<p>
It is now <%= @time %>
</p>
<p>
Time to say
<%= link_to "Goodbye!", :action => "goodbye" %>
</body>
</html>
http://localhost:3000/say/hello
Hello from Rails!
- Addition: 3
- Concatenation: cowboy
- Time in one hour: 2008-10-03 06:23:33 UTC
Ho!
Ho!
Ho!
Merry Christmas!
3...
2...
1...
Lift off!
Email: The <thekhuc@gmail.com>
It is now Fri Oct 03 01:23:33 -0400 2008
It is now Fri Oct 03 01:23:33 -0400 2008
Time to say Goodbye!
app\views\say\goodbye.html.erb
<html>
<head>
<title>See You Later!</title>
</head>
<body>
<h1>Goodbye!</h1>
<p>
It was nice having you here.
</p>
<p>
Time to say
<%= link_to "Hello!", :action => "hello" %>
</body>
</html>
http://localhost:3000/say/goodbye
Goodbye!
It was nice having you here.
Time to say Hello!
app\views\say\files.html.erb
<html>
<head>
<title>Files</title>
</head>
<body>
<ul>
<% for file in @files %>
<li>file name is <%= file %></li>
<% end %>
</ul>
</body>
</html>
http://localhost:3000/say/files
- file name is app
- file name is config
- file name is db
- file name is doc
- file name is lib
- file name is log
- file name is public
- file name is Rakefile
- file name is README
- file name is script
- file name is test
- file name is tmp
- file name is vendor
Wednesday, September 17, 2008
Agile Web Development with Rails - Third Edition
Friday, August 22, 2008
NAnt Intellisense in Visual Studio 2005
Kevin Miller has a great tutorial for getting Intellisense working for NAnt under Visual Studio 2005.
AnkhSVN and VS Command Shell
Add the following tools to your toolset if you're doing doing Continuous Integration with your .NET projects:
AnkhSVN - A Subversion SourceControl Provider for Visual Studio. The software allows you to perform the most common version control operations directly from inside the Microsoft Visual Studio IDE. With AnkhSVN you no longer need to leave your IDE to perform tasks like viewing the status of your source code, updating your Subversion working copy and committing changes. You can even browse your repository and you can plug-in your favorite diff tool.
VS Command Shell - VSCmdShell provides users with a shell window inside the Visual Studio IDE that can be used for Visual Studio commands as well. Current version allows user to use either Windows Command Shell (cmd.exe) or Windows PowerShell.
Friday, July 18, 2008
IIS 6 Fix for UrlRewriter.Net
Make sure you add a Wildcard application maps entry under Application Configuration for UrlRewriter.Net to work without file extensions, e.g. http://localhost/show/users.
Wednesday, July 16, 2008
Could Not Load File or Assembly Issue
Problem:
Could not load file or assembly 'System.EnterpriseServices, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a' or one of its dependencies. System could not find the file.
Cause:
System.EnterpriseServices.dll is missing in the GAC or there exist different versions of this dll.
Fix:
Drag and drop "<windows_directory>\Microsoft.NET\Framework\v2.0.50727\System.EnterpriseServices.dll"
into the "<windows_directory>\assembly\" folder.
Tuesday, July 15, 2008
Projects
Development Tools
NUnit
NUnit is a unit-testing framework for all .Net languages. Initially ported from JUnit, the current production release, version 2.4, is the fifth major release of this xUnit based unit testing tool for Microsoft .NET. It is written entirely in C# and has been completely redesigned to take advantage of many .NET language features, for example custom attributes and other reflection related capabilities. NUnit brings xUnit to all .NET languages.
NAnt
NAnt is a free .NET build tool. In theory it is kind of like make without make's wrinkles. In practice it's a lot like Ant. A
TortoiseSVN
Subversion client, implemented as a windows shell extension.
TortoiseSVN is a really easy to use Revision control / version control / source control software for Windows.
Since it's not an integration for a specific IDE you can use it with whatever development tools you like.
TortoiseSVN is free to use. You don't need to get a loan or pay a full years salary to use it.
DBVisualizer
DbVisualizer is a database tool useful for developers and database administrators to aid develop and maintain their databases. It is the perfect solution since the same tool can be used on all major operating systems accessing a wide range of databases.
Oracle Database 10g Express Edition
Oracle Database 10g Express Edition (Oracle Database XE) is a free, downloadable version of the world's most capable relational database.
Oracle Database XE is easy to install and easy to manage. With Oracle Database XE, you use the Database Home Page, an intuitive browser-based interface, to administer the database; create tables, views, and other schema objects; import, export, and view table data; run queries and SQL scripts; and generate reports.
Oracle Database XE includes Oracle HTML DB 2.1, a declarative, graphical development environment for creating database-centric Web applications. In addition to HTML DB 2.1, you can use popular Oracle and third-party languages and tools to develop your Oracle Database XE applications.
Links
http://www.nunit.org/index.php
http://nant.sourceforge.net/
http://tortoisesvn.tigris.org/
http://www.minq.se/products/dbvis/
http://www.oracle.com/technology/software/products/database/xe/index.html
Wednesday, June 25, 2008
Firefox 3 Broke My Code
Wednesday, June 18, 2008
Ray Allen is Back
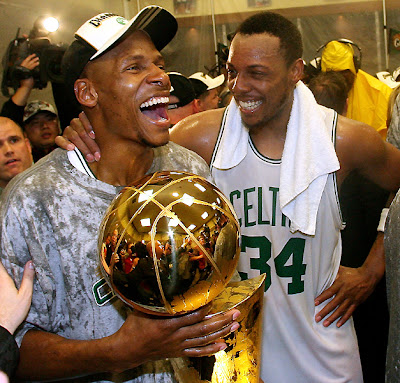
Source: NBA.COM
Friday, May 9, 2008
NBA Playoffs 2008
I started to watch the NBA Playoffs a few weeks ago, and I found the Jazz and the Hornets were fun to watch. The Jazz's #5 Carlos Boozer and #8 Deron Williams play great together, while the Hornets' #3 Chris Paul is becoming one of the League's best point guards. I'm rooting for the New Orleans Hornets to make it all way and win the 2008 NBA Playoffs.
Wednesday, May 7, 2008
Summer Has Arrived
I also took SWE 621 this semester. It's on Software Design and taught by Professor Robert Pettit. I learned to use IBM's Rational Software Architect software to design a racing system, which is a concurrent and real-time application. Got an opportunity to sharpen my UML skills as well as software design knowledge.
I would highly recommend both classes.
SWE 621 - This is a course in concepts and methods for the architectural design of software systems of sufficient size and complexity to require the effort of several people for many months. Fundamental design concepts and design notations are introduced. Several design methods are presented and compared, with examples of their use. Students will undertake a term project working in small groups addressing the design of a relatively complex software system.
SWE 637 - Concepts and techniques for testing software and assuring its quality. Topics cover software testing at the unit, module, subsystem, and system levels, automatic and manual techniques for generating and validating test data, the testing process, static vs. dynamic analysis, functional testing, inspections, and reliability assessment.
Friday, April 25, 2008
Wednesday, April 16, 2008
George Mason University Commencement 2008
2008 IT&E Convocation
When - Thursday, May 15, 2008
Time - 6 - 7:30 pm
Where - The Patriot Center - George Mason University
Speaker - Marc Willebeek-LeMair, Ph.D, Chief Technology Officer for 3Com
Schedule:
5:15pm Processional Preparation
5:30pm Processional
6:00pm Call to Order and Welcome
6:15pm Commencement Address
6:30pm Presentation of Diplomas
7:30pm Reception
Commencement 2008
When - Saturday May 17, 2008
Time - 10 am - noon
Where - The Patriot Center - George Mason University
The 41st annual George Mason University commencement is scheduled for Saturday, May 17th, 2008 at 10:00 a.m. in the Patriot Center. The complete schedule of all graduation ceremonies will be online. Graduates are requested to arrive at the North Plaza, near the George Mason statue, for assembly promptly at 9:00 a.m. on May 17th. Patriot Center doors open for guests at 9:00 a.m. The ceremony begins at 10:00 a.m. and lasts approximately two hours.
Monday, April 14, 2008
Monday, March 31, 2008
Must-Learned Semantic Tools
Semantic Web/RDF Library for C#/.NET
SemWeb is my Semantic Web/RDF library written in C# for Mono or Microsoft's .NET 1.1/2.0. The library can be used for reading and writing RDF (XML, N3), keeping RDF in persistent storage (memory, MySQL, etc.), querying persistent storage via simple graph matching and SPARQL, and making SPARQL queries to remote endpoints. Limited RDFS and general-purpose inferencing is also possible. SemWeb's API is straight-forward and flexible.
ASP.NET RSS Toolkit
RssDataSource control to consume feeds in ASP.NET applications:
- Works with ASP.NET data bound controls
- Implements schema to generate columns at design time
- Supports auto-generation of columns at runtime (via ICustomTypeDescriptor implementation)
Caching of downloaded feeds both in-memory and on-disk (persisted across process restarts)
Generation of strongly typed classes for feeds (including strongly typed channel, items, image, handler) based on a feed URL (the toolkit recognizes RSS, Atom and RDF feeds) or a file containing a sample feed. Allows programmatically download (and creation) of feeds using strongly-typed classes.
LinqToRdf
LinqToRdf provides a full-featured LINQ query provider for .NET using both local triple stores with Graph Matching and SPARQL queries on remote stores. It also provides graphical design tools for visual studio 2008 that provide a UML style design surface for the production of both ontologies and .NET domain models.
Thursday, March 13, 2008
Voiceless Future
Thursday, February 28, 2008
Monday, February 25, 2008
Wednesday, February 20, 2008
Relatives Visit and Other Stuff
Wednesday, February 6, 2008
Happy Vietnamese New Year 2008
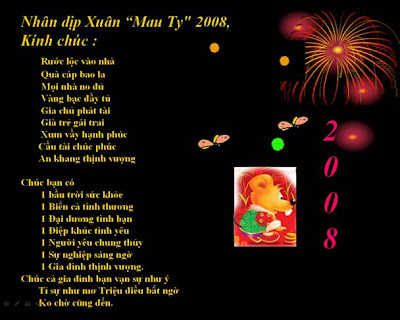
My literal translation:
Wishes for the new year:
Bring luck into the house
Plenty of gifts
A full house
A drawer full of jewelries
Prosperous household
Old and young
Together and blessed
Wealthy and happy
Safe and prosper
Wishing you:
1 Sky filled with health
1 Sea filled with love
1 Ocean filled with friendship
1 Melody filled with love
1 Faithful lover
1 Bright career
1 Prosperous family
Wishing everyone a new year filled with wonderful dreams and surprises.
Monday, January 28, 2008
January Birthdays
Friday, January 25, 2008
Microsoft Silverlight for Developers
Date/Time: | Wednesday, January 30, 2008 6:00 PM - Wednesday, January 30, 2008 8:00 PM |
Language(s): | English. |
Product(s): | Other |
Audience(s): | Architect,Developer,Other |
Event Overview
Abstract
Microsoft® Silverlight™ is a cross-browser, cross-platform plug-in for delivering the next generation of .NET based media experiences and rich interactive applications for the Web. Silverlight offers a flexible programming model that supports AJAX, VB, C#, Python, and Ruby, and integrates with existing Web applications. Silverlight supports fast, cost-effective delivery of high-quality video to all major browsers running on the Windows , Mac OS, and Linux coming soon.
Who Should Attend
Architects, Developers, and anyone who would like to learn how to take their AJAX applications to the next level .
What you will learn
· The benefits of Silverlight from a developer perspective
· How to build Silverlight 1.0 applications using JavaScript & AJAX programming NOW
· How UI designers and developers can collaborate using the same codebase, but with role specific tools
· How Silverlight 2.0 (the next version) brings a subset of the Windows Presentation Foundation (WPF) & .NET Framework cross platform / cross browser
· Fundamentals of building Silverlight 2.0 applications using C# & the .NET Framework
· How the ASP.NET 3.5 Extensions make building Silverlight solutions easier (Think cross platform video by simply dragging/dropping a control + pointing to a video file)
Pete Brown, from Applied Information Sciences (AIS), will be closing the dinner by sharing his experiences & lessons learned from building a production Silverlight application.
Note: This will be a developer-centric talk with a heavy focus on code demos.
Wednesday, January 23, 2008
Date and Time Format Patterns
Date and Time Format Patterns
All the patterns:
0 MM/dd/yyyy 01/23/2008
1 dddd, dd MMMM yyyy Wednesday, 23 January 2008
2 dddd, dd MMMM yyyy HH:mm Wednesday, 23 January 2008 16:38
3 dddd, dd MMMM yyyy hh:mm tt Wednesday, 23 January 2008 04:38 PM
4 dddd, dd MMMM yyyy H:mm Wednesday, 23 January 2008 16:38
5 dddd, dd MMMM yyyy h:mm tt Wednesday, 23 January 2008 4:38 PM
6 dddd, dd MMMM yyyy HH:mm:ss Wednesday, 23 January 2008 16:38:03
7 MM/dd/yyyy HH:mm 01/23/2008 16:38
8 MM/dd/yyyy hh:mm tt 01/23/2008 04:38 PM
9 MM/dd/yyyy H:mm 01/23/2008 16:38
10 MM/dd/yyyy h:mm tt 01/23/2008 4:38 PM
11 MM/dd/yyyy HH:mm:ss 01/23/2008 16:38:03
12 MMMM dd January 23
13 MMMM dd January 23
14 yyyy'-'MM'-'dd'T'HH':'mm':'ss.fffffffK 2008-01-23T16:38:03.1183581-05:00
15 yyyy'-'MM'-'dd'T'HH':'mm':'ss.fffffffK 2008-01-23T16:38:03.1183581-05:00
16 ddd, dd MMM yyyy HH':'mm':'ss 'GMT' Wed, 23 Jan 2008 16:38:03 GMT
17 ddd, dd MMM yyyy HH':'mm':'ss 'GMT' Wed, 23 Jan 2008 16:38:03 GMT
18 yyyy'-'MM'-'dd'T'HH':'mm':'ss 2008-01-23T16:38:03
19 HH:mm 16:38
20 hh:mm tt 04:38 PM
21 H:mm 16:38
22 h:mm tt 4:38 PM
23 HH:mm:ss 16:38:03
24 yyyy'-'MM'-'dd HH':'mm':'ss'Z' 2008-01-23 16:38:03Z
25 dddd, dd MMMM yyyy HH:mm:ss Wednesday, 23 January 2008 16:38:03
26 yyyy MMMM 2008 January
27 yyyy MMMM 2008 January
The patterns for DateTime.ToString ( 'd' ) :
0 MM/dd/yyyy 01/23/2008
The patterns for DateTime.ToString ( 'D' ) :
0 dddd, dd MMMM yyyy Wednesday, 23 January 2008
The patterns for DateTime.ToString ( 'f' ) :
0 dddd, dd MMMM yyyy HH:mm Wednesday, 23 January 2008 16:38
1 dddd, dd MMMM yyyy hh:mm tt Wednesday, 23 January 2008 04:38 PM
2 dddd, dd MMMM yyyy H:mm Wednesday, 23 January 2008 16:38
3 dddd, dd MMMM yyyy h:mm tt Wednesday, 23 January 2008 4:38 PM
The patterns for DateTime.ToString ( 'F' ) :
dddd, dd MMMM yyyy HH:mm:ss Wednesday, 23 January 2008 16:38:03
The patterns for DateTime.ToString ( 'g' ) :
0 MM/dd/yyyy HH:mm 01/23/2008 16:38
1 MM/dd/yyyy hh:mm tt 01/23/2008 04:38 PM
2 MM/dd/yyyy H:mm 01/23/2008 16:38
MM/dd/yyyy h:mm tt 01/23/2008 4:38 PM
The patterns for DateTime.ToString ( 'G' ) :
MM/dd/yyyy HH:mm:ss 01/23/2008 16:38:03
The patterns for DateTime.ToString ( 'm' ) :
MMMM dd January 23
The patterns for DateTime.ToString ( 'r' ) :
ddd, dd MMM yyyy HH':'mm':'ss 'GMT' Wed, 23 Jan 2008 16:38:03 GMT
The patterns for DateTime.ToString ( 's' ) :
yyyy'-'MM'-'dd'T'HH':'mm':'ss 2008-01-23T16:38:03
The patterns for DateTime.ToString ( 'u' ) :
yyyy'-'MM'-'dd HH':'mm':'ss'Z' 2008-01-23 16:38:03Z
The patterns for DateTime.ToString ( 'U' ) :
dddd, dd MMMM yyyy HH:mm:ss Wednesday, 23 January 2008 16:38:03
The patterns for DateTime.ToString ( 'y' ) :
yyyy MMMM 2008 January